forked from grumpydevelop/federator
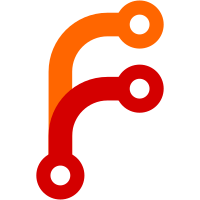
- permissions are always lower-case - revert webfinger changes to initial state - re-add setFirst and setLast for outbox - we now save the current host (f.e. https://contentnation.net) to save users with their respective host (a request to fedusers/grumpydevelop will then result in saving the user as grumpydevelop@contentnation.net)
97 lines
2.6 KiB
PHP
97 lines
2.6 KiB
PHP
<?php
|
|
/**
|
|
* SPDX-FileCopyrightText: 2024 Sascha Nitsch (grumpydeveloper) https://contentnation.net/@grumpydevelop
|
|
* SPDX-License-Identifier: GPL-3.0-or-later
|
|
*
|
|
* @author Sascha Nitsch (grumpydeveloper)
|
|
**/
|
|
|
|
namespace Federator\Api\FedUsers;
|
|
|
|
/**
|
|
* handle activitypub outbox requests
|
|
*/
|
|
class Outbox implements \Federator\Api\FedUsers\FedUsersInterface
|
|
{
|
|
/**
|
|
* main instance
|
|
*
|
|
* @var \Federator\Main $main
|
|
*/
|
|
private $main;
|
|
|
|
/**
|
|
* constructor
|
|
* @param \Federator\Main $main main instance
|
|
*/
|
|
public function __construct($main)
|
|
{
|
|
$this->main = $main;
|
|
}
|
|
|
|
/**
|
|
* handle get call
|
|
*
|
|
* @param string $_user user to fetch outbox for
|
|
* @return string|false response
|
|
*/
|
|
public function get($_user)
|
|
{
|
|
$dbh = $this->main->getDatabase();
|
|
$cache = $this->main->getCache();
|
|
$connector = $this->main->getConnector();
|
|
|
|
// get user
|
|
$user = \Federator\DIO\User::getUserByName(
|
|
$dbh,
|
|
$_user,
|
|
$connector,
|
|
$cache
|
|
);
|
|
if ($user->id === null) {
|
|
return false;
|
|
}
|
|
|
|
// get posts from user
|
|
$outbox = new \Federator\Data\ActivityPub\Common\Outbox();
|
|
$min = $this->main->extractFromURI("min", "");
|
|
$max = $this->main->extractFromURI("max", "");
|
|
$page = $this->main->extractFromURI("page", "");
|
|
if ($page !== "") {
|
|
$items = \Federator\DIO\Posts::getPostsByUser($dbh, $user->id, $connector, $cache, $min, $max);
|
|
$outbox->setItems($items);
|
|
} else {
|
|
$items = [];
|
|
}
|
|
$host = $_SERVER['SERVER_NAME'];
|
|
$id = 'https://' . $host .'/' . $_user . '/outbox';
|
|
$outbox->setPartOf($id);
|
|
$outbox->setID($id);
|
|
if ($page !== '') {
|
|
$id .= '?page=' . urlencode($page);
|
|
}
|
|
if ($page === '' || $outbox->count() == 0) {
|
|
$outbox->setFirst($id);
|
|
$outbox->setLast($id . '&min=0');
|
|
}
|
|
if (sizeof($items)>0) {
|
|
$newestId = $items[0]->getPublished();
|
|
$oldestId = $items[sizeof($items)-1]->getPublished();
|
|
$outbox->setNext($id . '&max=' . $newestId);
|
|
$outbox->setPrev($id . '&min=' . $oldestId);
|
|
}
|
|
$obj = $outbox->toObject();
|
|
return json_encode($obj, JSON_UNESCAPED_SLASHES | JSON_PRETTY_PRINT);
|
|
}
|
|
|
|
/**
|
|
* handle post call
|
|
*
|
|
* @param string $_user user to add data to outbox @unused-param
|
|
* @return string|false response
|
|
*/
|
|
public function post($_user)
|
|
{
|
|
return false;
|
|
}
|
|
}
|